A very frequent question that gets asked on all forums is How do I slice an array or a list or a tuple in Python? So I thought maybe I can write a post to help to answer this question in a comprehensive manner.
As the name suggests Slice in python takes out slices from the list or tuple and puts it in another list or tuple respectively.
“Yes you can slice a String as well!” And guess what it returns a string as the output 🙂
According to the Python, documentation Slice has the following syntax
Syntax
Slice(start, end, step) |
Parameters
Parameter | Description |
start | Start position for slicing. It is optional and the default is 0 (zero) |
end | End position for slicing. Not optional |
step | Step for the slicing. It is optional and the default is 1 |
Few notes before we continue,
- It should be easy to understand start position as we define the positions in an array starting at 0
- The end position is just a count, not a position in the array (we will see it happening in some time!)
- Step and end position can go negative as well (wait and watch 🙂 )
- Default direction for the slice is from left to right BUT you can go from right to left as well
With slice you don’t always have to begin at the beginning you begin from the end as well! So start can be end and the end can be start just make sure to jump backwards 🙂
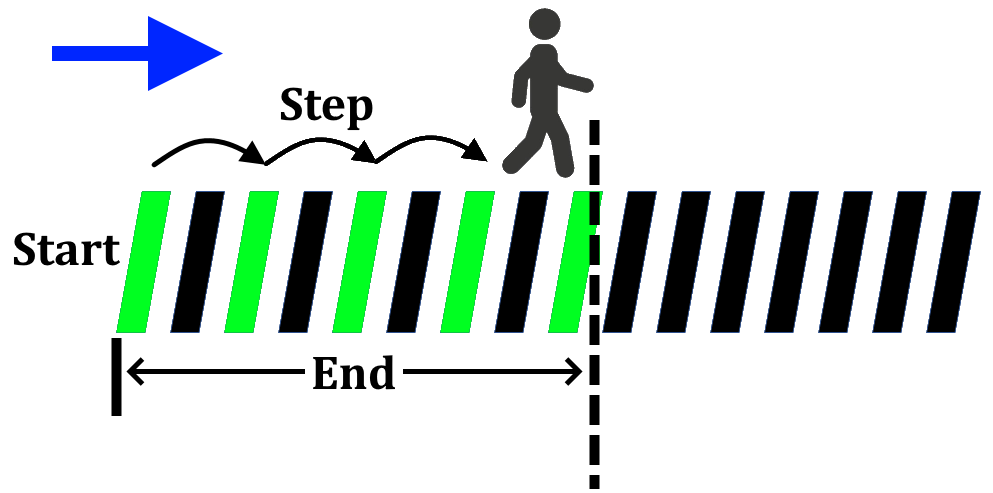
Let’s Slice a Bit!
Let’s take a look at the following the code. I have taken a simple tuple for understanding purpose
a = (1,2,3,4,5,6,7,8,9,10) slice1 = slice(0,10,1) # Simple slice slice2 = slice(0,10,2) # Slice to get alternate number slice3 = slice(1,7) # Slice the tuple without any steps slice4 = slice(2,9,3) # Start at a random place end at random place and have step 3 print(a[slice1]) # Outputs (1,2,3,4,5,6,7,8,9,10) print(a[slice2]) # Outputs (1, 3, 5, 7, 9) print(a[slice3]) # Outputs (2, 3, 4, 5, 6, 7) print(a[slice4]) # Outputs (3, 6, 9)
Now, let’s take a U-Turn. Let’s begin at the end.
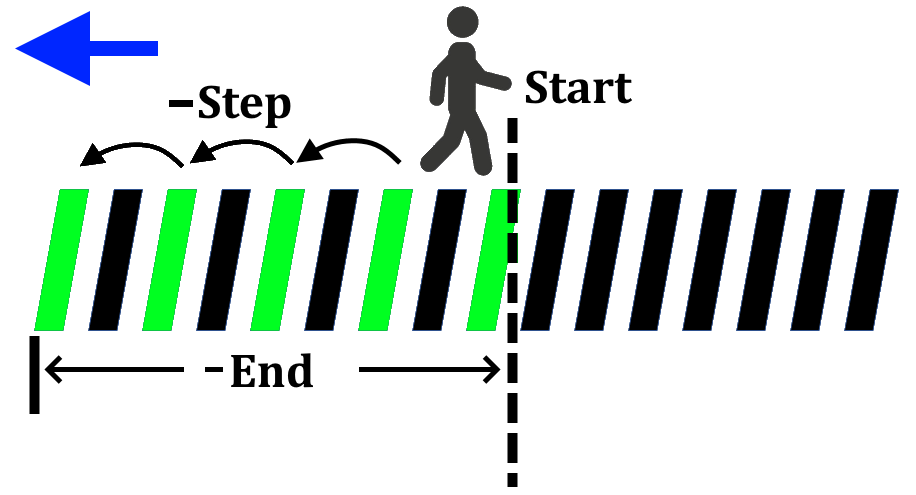
Till now we began at the beginning of the list ie left side of the list and loved towards the end ie towards the right. Now, let’s try to ‘Slice’ the list from the end of the list moving towards the beginning of the list.
The first step towards taking this U-Turn is to set the start position as the end position and move towards the left or beginning and we will put the step on reverse direction.
Please take a look at the following code. I have kept the same simple tuple for understanding purpose
a = (1,2,3,4,5,6,7,8,9,10) slice1 = slice(9,-11,-1) print(a[slice1]) # Outputs (10, 9, 8, 7, 6, 5, 4, 3, 2, 1)
Here, we defined slice(9,-11,-1)
- The Start position is 9 ie the last element in the tuple.
- The End position is -11. The negative sign ‘-‘ indicates that the slicer will move in the opposite direction ie right to left.
- The Step is -1. Again the negative sign ‘-‘ indicates that the slicer will take the step backward while slicing.
Try running the following code in Python and check what is the output?
a = (1,2,3,4,5,6,7,8,9,10) slice1 = slice(9,-11,-2)
Extended Slice
Do we really have to call the method slice()?
I have good news. The answer is NO. You can use extended slice syntax to quickly slice an array, list, tuple or a string.
Here’s how you do it
a = (1,2,3,4,5,6,7,8,9,10) b = a[0:10:1] print(b) # Outputs (1, 2, 3, 4, 5, 6, 7, 8, 9, 10) c = a[9:-11:-1] print(c) # Outputs (10, 9, 8, 7, 6, 5, 4, 3, 2, 1)
Pretty cool right? So here’s what happened
- We used the same start, end, and step but, within ‘[]’ instead of using slice()
- We used colons’ ‘:’ instead of comma ‘,’
- Rest of the Syntax remained the same!
a[slice(0,10,1)] -> a[0:10:1] |
a[slice(9,-11,-1)] -> a[9:-11:-1] |
So, I have tried to lay down the basics of Slice method. Please give it a try and let me know what you found?
Don’t forget to check another post where I have added examples of slice() and extended slice..
Happy Coding 🙂
4 Replies to “How to Slice an Array in Python?”